When it comes to the development of websites, JavaScript is the language for many of the developers worldwide. Among all the duties developers have to solve, one of the most frequent is changing stars. For form validation, data processing, or just simple text displaying, proper utilization of strings is always a compelling job in JavaScript. In this blog post, I will explain all methods of javascript string manipulation and provide you with code samples to work with.
Table of Contents
Learn the basics of working with strings in JavaScript
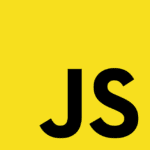
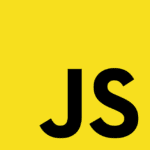
In JavaScript, a string is a sequence of characters to represent text. It can be formatted with single quotes, double quotes, or a backslash. Here is a brief example.
let singleQuoteString = ‘Hello, World!’;
let doubleQuoteString = “Hello, World!”;
let backtickString = `Hello, World! `;
Basic String Methods
length
The length property is used to get the number of characters that are in a given string.
let str = ‘Hello, World!’;
console. log(str. length); // Output: 13
charAt()
The charAt() method returns the character present at the given index position.
let str = "Hello, World!";
console.log(str.charAt(0)); // Output: H
indexOf()
The indexOf() method allows finding the first occurrence of a certain value in the string.
let str = "Hello, World!";
console.log(str.indexOf('o')); // Output: 4
slice()
The slice() method cuts a portion of a string and returns the cut part as a new string.
let str = "Hello, World!";
console.log(str.slice(0, 5)); // Output: Hello
substring()
The substring() method works like slice() but it does not accept negative values of indices.
let str = "Hello, World!";
console.log(str.substring(0, 5)); // Output: Hello
substr()
The substr() method is used to cut a part of the string starting from a specific index and till the specified number of characters.
let str = "Hello, World!";
console.log(str.substr(0, 5)); // Output: Hello
Advanced String Methods
replace()
The replace() method scans a string for a specific element and then returns a new string containing the element replaced.
let str = "Hello, World!";
let newStr = str.replace('World', 'JavaScript');
console.log(newStr); // Output: Hello, JavaScript!
split()
With the help of the split() method, a string is divided into an array of substrings.
let str = "Hello, World!";
let arr = str.split(', ');
console.log(arr); // Output: ["Hello", "World!"]
concat
The concat() method concatenates (joins) two or more strings.
let str1 = "Hello";
let str2 = "World";
let result = str1.concat(', ', str2, '!');
console.log(result); // Output: Hello, World!
toUpperCase() and toLowerCase()
The toUpperCase() method converts a string to uppercase letters, while toLowerCase() converts a string to lowercase letters.
let str = "Hello, World!";
console.log(str.toUpperCase()); // Output: HELLO, WORLD!
console.log(str.toLowerCase()); // Output: hello, world!
trim()
The trim() method removes whitespace from both ends of a string.
let str = " Hello, World! ";
console.log(str.trim()); // Output: Hello, World!
Template Literals
Template literals, introduced in ES6, provide an easy way to work with strings. They allow for embedding expressions and multi-line strings, using backticks (`).
let name = "JavaScript";
let greeting = `Hello, ${name}!`;
console.log(greeting); // Output: Hello, JavaScript!
let multiLineStr = `This is a string
that spans multiple
lines.`;
console.log(multiLineStr);
/* Output:
This is a string
that spans multiple
lines.
*/
Regular Expressions
Regular expressions (regex) are patterns used to match character combinations in strings. They are incredibly powerful for searching and replacing text.
let str = "Hello, World!";
let regex = /World/;
console.log(str.match(regex)); // Output: ["World"]
let newStr = str.replace(/World/, 'JavaScript');
console.log(newStr); // Output: Hello, JavaScript!
Example: Email Validation
Here is an example of how the regex will look like in checking the validity of an email address.
function validateEmail(email) {
let regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
console.log(validateEmail('test@example.com')); // Output: true
console.log(validateEmail('invalid-email')); // Output: false
Conclusion
Operations involving strings are the most basic operations that can be performed during JavaScript String Manipulation. From the simplest ones, such as length, charAt(), and slice to more complex ones involving regex and template literals, one’s capability of utilizing these tools enhances the likelihood of their optimized work and efficiency. We surely expect that the above mentioned guide has helped you lay down the basic foundation to manipulate strings in JavaScript. Happy coding!
References
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String
- https://www.w3schools.com/js/js_string_methods.asp
[…] DNS Servers: Finally, these servers know precisely where https://www.nexgismo.com lives and give its IP […]